Welcome to the world of Python – a versatile, open-source, multi-paradigm programming language that simply loves data and metrics. Python stands out with its simplicity and readability, and these characteristics make it an ideal tool, especially for budding programmers.
In the modern business world, data analysis is no longer a luxury but a significant necessity. The ability to sift through vast amounts of data, analyze them for actionable insights, and make informed decisions can be a game changer for businesses.
So, from where does Python fit into this picture? One word: simplicity. Python’s clean and efficient syntax, along with its powerful libraries like Pandas, NumPy, and Matplotlib, make it a top choice for handling intricate tasks of data analysis. It makes performing complex data manipulations, modeling data, and creating beautiful visualizations a breeze.
In this blog post, we will dive deep into the Python language and its libraries, and showcase its might in the world of data analysis. Whether you’re a data enthusiast or a seasoned analyst, get ready for an exciting journey in the Python landscape!
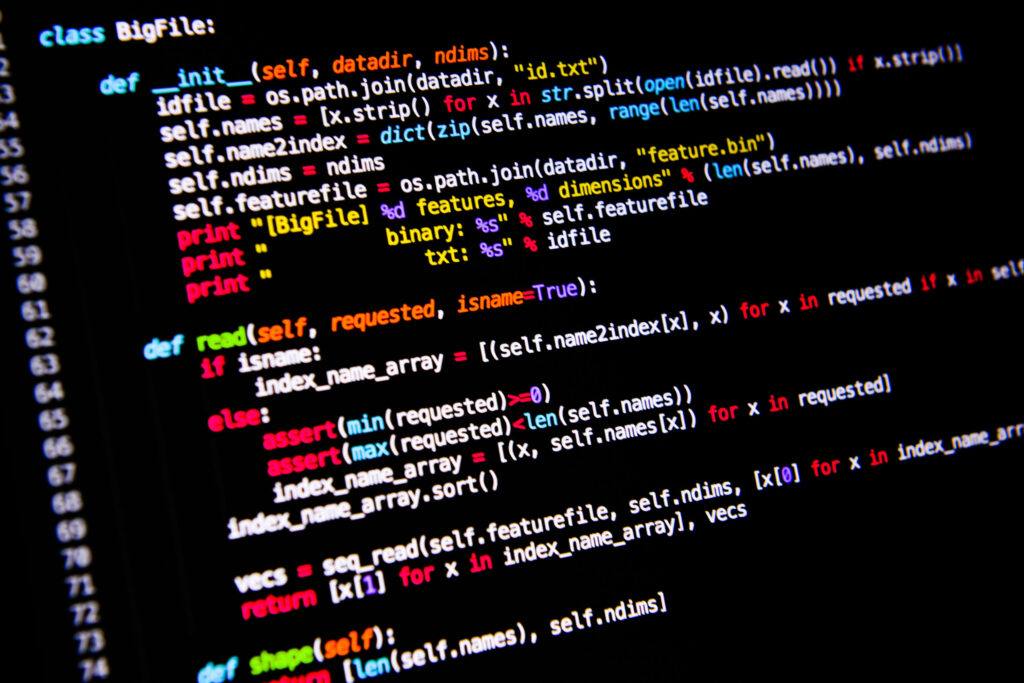
Python Basics for Data Analysis
Before we dive deep into how Python can be harnessed for data analysis, it is crucial we understand some Python basics – Data Structures and Python Libraries specifically designed for data analysis.
Python Data Structures
The main data structures in Python include Lists, Tuples, Dictionaries, and Sets. These are vital because they offer various ways to organize data in a program.
- Lists and Tuples are used for storing a sequence of values.
- Dictionaries, on the other hand, are excellent for pairing keys with values; it’s similar to a mini database.
- Sets help in storing non-repeated elements.
These data structures play an integral role when analyzing data.
Python Libraries for Data Analysis
Python boasts numerous libraries custom-made for data analysis. The top three picks, revered in the world of data analysis, are:
- Pandas: This is your data analysis workhorse. It provides data structures and functions essential for manipulating structured data.
- NumPy: This library is notable for mathematical computations. It extends lists to multi-dimensions and provides numerical arrays. Perfect for linear algebra and Fourier transform operations.
- Matplotlib: This is the go-to library for data visualization in Python. It helps create charts and graphs.
Mastering these basics sets the ground for successful data analysis with Python.
Data Analysis with Python: A Comprehensive Walkthrough
Embarking on a journey into the world of data analysis using Python? You’ve chosen a great companion, as Python, with its extensive libraries and community support, is a favorite of many data enthusiasts. This guide will navigate you through the stages, ensuring you have a smooth ride.
1. Acquiring the Dataset
Every analysis starts with data. Where do you find it?
- Open-source platforms: Websites such as Kaggle and the UCI Machine Learning Repository host an abundant variety of datasets spanning numerous domains and research areas.
2. Importing and Reading the Dataset with Pandas
Before any analysis, you need to load your data into Python:
import pandas as pd
# Load the dataset
data = pd.read_csv('path_to_your_dataset.csv')
This command reads a CSV file into a Pandas DataFrame, a versatile data structure that makes data manipulation a breeze.
3. Cleaning and Preprocessing the Data
Real-world data is rarely perfect. It often contains missing values, outliers, or inconsistencies.
# Check for missing values
missing_values = data.isnull().sum()
# Drop rows with missing values
cleaned_data = data.dropna()
# Alternatively, fill missing values with mean (or other methods)
filled_data = data.fillna(data.mean())
This step ensures the quality and reliability of your subsequent analysis.
4. Exploring and Analyzing the Data
With a clean dataset in hand, you can start uncovering insights:
# Get a summary of the data
summary = data.describe()
# Find correlation between variables
correlation_matrix = data.corr()
These commands give you a statistical summary and a correlation matrix, respectively, offering a birds-eye view of your data’s characteristics.
5. Visualizing Data with Matplotlib and Seaborn
Visualization enhances the understanding of data patterns and relationships:
import matplotlib.pyplot as plt
import seaborn as sns
# A basic histogram
plt.hist(data['column_name'])
plt.show()
# A more complex Seaborn pairplot
sns.pairplot(data, hue='categorical_column_name')
plt.show()
With Matplotlib and Seaborn, you can create a plethora of visualizations, from basic plots to intricate multi-variable graphs.
Diving into data analysis with Python is both rewarding and exhilarating. With the vast tools and libraries at your disposal, each analysis becomes a journey of discovery. Happy analyzing!
Exploring Python Libraries in Detail
Deep Dive Into Pandas
Pandas is a crucial library for Python data analysis. Its primary structures, Series and DataFrames, are exceedingly practical for data manipulation. A Series is a one-dimensional array, while a DataFrame is a two-dimensional table. This permits easy management of large datasets.
Another core feature of Pandas is data aggregation and grouping. With this, you can group data based on certain conditions and perform calculation operations on those groups. Imagine the possibilities!
Deep Dive Into NumPy
NumPy, fundamental for numerical computations, has at its core, the ndarray object: a homogenous, multidimensional array allowing complex operations and manipulations. Noteworthy too are the mathematical and statistical methods in NumPy, such as mathematical operations on arrays, descriptive statistics, and random number generations.
Deep Dive Into Matplotlib
Last but definitely not least, Matplotlib allows us to bring our data to life with visualizations. We can start with basic plotting, which presents numerical data in a graphical format. For a more complex representation, histograms and scatter plots work wonders in displaying data patterns.
In summary, these libraries combined create the powerful toolset Python offers for data analysis. Time to dive into these deep waters!
Absolutely! Here’s a more detailed and comprehensive rewrite of the section:
Diving Deeper: Advanced Data Analysis Techniques with Python
Once you’re comfortable with Python’s basic data analysis techniques, a universe of advanced methodologies awaits. Two crucial pillars in this advanced realm are Correlation & Regression and Time Series Analysis.
1. Correlation and Regression: Decoding Relationships
Understanding individual data distributions is foundational, but the real magic happens when you start examining the intricate relationships between data attributes.
Correlation:
This statistical measure indicates the degree to which two variables move in relation to each other:
import pandas as pd
# Load the dataset
data = pd.read_csv('path_to_your_dataset.csv')
# Calculate correlation matrix
correlation_matrix = data.corr()
print(correlation_matrix)
Regression:
Beyond merely identifying relationships, regression assists in predicting and forecasting trends:
import statsmodels.api as sm
# Assume 'X' as independent and 'Y' as dependent variables
X = data['X_column_name']
Y = data['Y_column_name']
X = sm.add_constant(X) # Adds a constant (intercept) to the model
model = sm.OLS(Y, X).fit() # Ordinary Least Squares regression model
predictions = model.predict(X)
print(model.summary())
2. Time Series Analysis: Tracking Changes Over Time
Time Series Analysis helps unravel patterns in data sequences collected over intervals, making it vital for forecasting:
# Converting a column to datetime format in pandas
data['date_column_name'] = pd.to_datetime(data['date_column_name'])
# Setting the date column as the index
data.set_index('date_column_name', inplace=True)
# Resampling data to get monthly averages
monthly_data = data.resample('M').mean()
With pandas
, handling time-series data becomes incredibly straightforward. For more advanced operations, like ARIMA (AutoRegressive Integrated Moving Average) models, Python offers libraries like statsmodels
.
Exploring these advanced avenues amplifies your analytical prowess, unveiling deeper insights from your data. Python, with its rich ecosystem, ensures that your analytical journey is both enlightening and exhilarating. Dive in and let the data reveal its secrets!
Real-world Examples of Data Analysis Using Python
Let’s delve into how Python comes to life in practical scenarios. We’ll explore two diverse sectors – sales and healthcare, and analyze how Python aids in dissecting data for these fields.
Sales Data Analysis with Python
Imagine you’re dealing with a gargantuan pool of sales data. Sifting through it manually? Nope! Enter Python. Let’s consider an e-commerce setup. Here, Python comes in handy for tasks like:
- Identifying trends and patterns in sales.
- Evaluating the performance of a particular product over time.
- Comparing sales across different regions.
By dissecting this data, Python allows businesses to target problem areas and optimize strategies, enhancing sales performance.
Deep-Dive: HealthCare Data Analysis
Next, let’s jump into the healthcare arena. With Python, healthcare professionals can:
- Use patient history to predict possible future illnesses.
- Identify risk factors contributing to diseases.
- Improve healthcare service delivery by analyzing patient feedback.
Python’s data analysis capabilities allow healthcare professionals to draw inferences that aid in better patient care, diagnosis, treatment, and overall service.
To conclude, Python’s prowess is unmatched when it comes to data analysis, as demonstrated by real-world examples from varied sectors.
Conclusion
In our journey to understand the significance of Python in Data Analysis, we’ve unraveled its immense potential and flexibility as a tool. Python, with its user-friendly syntax, vast library ecosystem, and powerful data manipulation capabilities, stands tall as a leading language in the Data Analysis domain.
Re-emphasizing the Importance of Python in Data Analysis
Python shines in Data Analysis. From handling large datasets, cleaning, and preprocessing data, to exploratory analysis and creating interactive visualizations, Python has proven its efficiency. Industries worldwide rely on it to glean insights from their data and make informed business decisions.
Future Trends in Data Analysis
Data analysis isn’t a stagnant field, it’s dynamic and ever-evolving. As we leap into the future, Trends such as Machine Learning, Artificial Intelligence, and Predictive Analysis are set to redefine the way we understand and interpret data. And guess what? Python is a front-runner with robust support for these rising trends.
Whether you are a newbie venturing into data analysis, a seasoned data scientist, or an organization driven by data, Python is a powerful ally you’d want to have on your side. When it’s about data analysis, dig into Python!